Dear Lifehacker, I have some background in coding, but I’ve never tried Android development before. I’d like to get started, but I’m not entirely sure what I need. I don’t need to “learn to code” per se, but I could use some guidance on where to start with Android. Can you help? Thanks, Dreaming of Electric Sheep
Dear Mr. K. Dick,
As you’re probably aware, writing apps for Android is more than just learning code syntax. However, there are still a whole host of tools and resources you might not be familiar with that you may need to make Android apps.
Note: this is not meant to be a comprehensive guide with every detail of these applications and resources. In fact, such a guide would require a book-length piece. However, we will give you an overview of the different tools you can use and where to find more information. These tools require varying levels of experience. However, if you’re ready to move from theory and syntax to actual development, here’s what you’ll need.
The Android Software Development Kit (SDK)
The Android Software Development Kit (SDK) is actually a collection of tools that will help you make Android apps. There’s more outside the SDK that we’ll discuss, but here are some of the most helpful tools in the SDK:
Eclipse/Android Studio
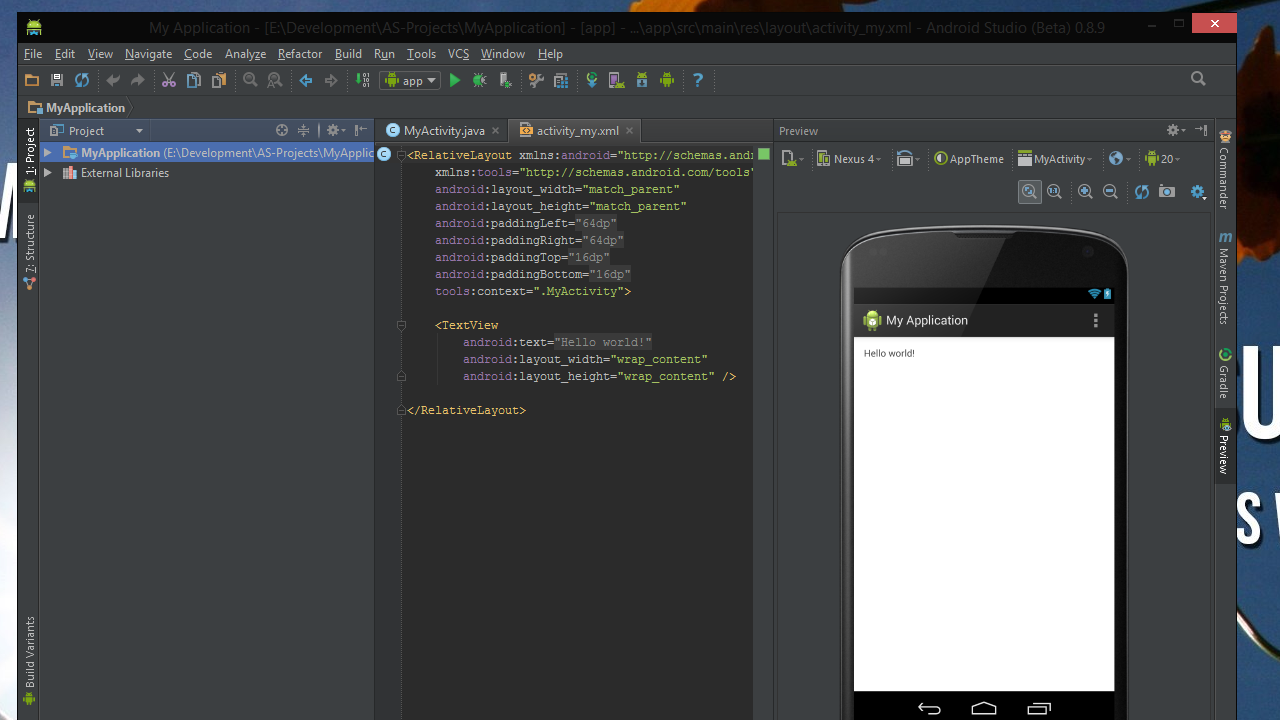
There are two primary integrated development environments (IDE) for Android. An IDE is the main program where you’ll write code and put your app together. It can help you organise and edit the various files in your app, manage the packages and supporting libraries you app will need, and test it out on real devices or emulators.
The default IDE for Android is Eclipse. Eclipse allows you to modify Java and XML files and organise the various pieces of your application, among many other tasks. The version you get from Google also includes a package manager that allows you to update to the latest version of Android tools as soon as Google releases them.
The main alternative is Android Studio, which is currently being made directly by Google. Like many Google projects, Android Studio is part of a prolonged beta. The long-term intention is for Android Studio to replace Eclipse as the primary IDE for Android development. That doesn’t necessarily mean it’s for everyone. For example, if you need to make use of the Native Development Kit for apps like games, Eclipse is mandatory. However, Android Studio is a good option if you want to get a jump start on the future, and you’re willing to tolerate some possible bugs.
No matter which IDE you choose, using it is a bit like Photoshop: it can do a lot of cool things, but you’ll probably only learn the individual tools as you need them. However, this is also a good place to get started on some of the basics of Android development. Here are some great tutorials and resources to get you started:
- Udacity – Developing Android Apps: This 8-week online class has a good amount of free elements, taught directly by Google engineers. The course won’t just copy-paste code, but it will help you learn some of the core concepts and features you’ll need.
- Android Developer Training: Part of Google’s documentation includes training tutorials on how to use its tools. These documents will walk you through basic features of the IDE. If you don’t have much experience developing applications, this might not turn you into a master dev, but it will help you learn the tools.
- Vogella: It’s worth mentioning Vogella tutorials in just about every section here. This massive set of tutorials covers just about everything you could cover. If you have a basic question not covered above, check Vogella.
ADB
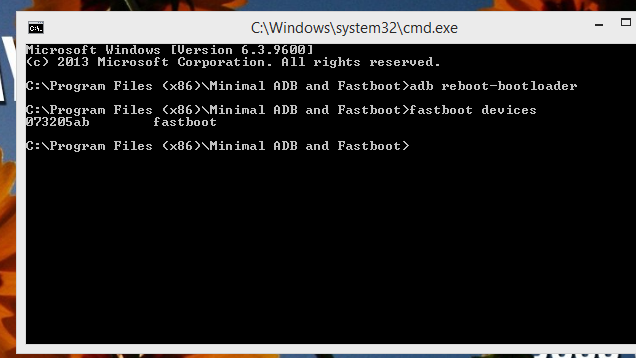
We’ve talked about ADB before from a regular user perspective, but the tool’s primary purpose is actually to aid in development. As such, it’s included in the Android SDK. You can use this to load software or make changes to your devices when it’s plugged into your computer. We’ve described the basic tools you can use with ADB, but if you want to learn more as a developer, check these out:
- ADB Documentation: This is the primary resource from Google on what ADB is and how it works. You can find most of what ADB is capable of here.
- Vogella – Using the Android Debug Bridge: Another Vogella tutorial, this one covers the basics of how ADB works and some of the common things you can do with it. If you don’t want to dig through Google’s documentation for the one command you need, this might be a good place to start.
Android Developer Guidelines
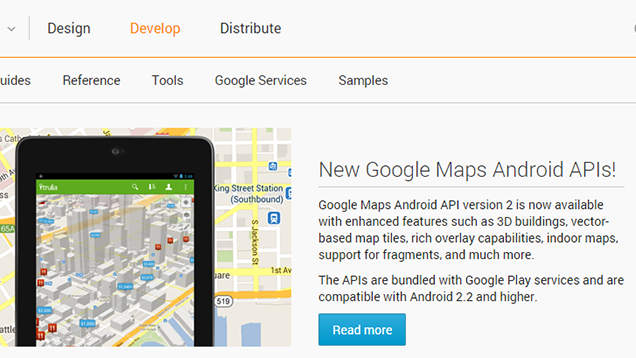
We’ve already linked to a couple of resources from the official Android Developer Guidelines so far, which only proves how useful they are. Google maintains a vast, extensive collection of documentation and resources for how to program your apps that you can reference or search through.
If you’re brand new to Android development, it can’t hurt to browse through some of the tutorials and guides here. They’re laid out in such a way that one lends into another (see the Android Developer Training above). Here are some sections that are worth brushing up on if you’re getting started:
- Google Services: We’ve talked about Google Play Services before, but here’s where you get to see what’s going on under the hood. Google offers a wide variety of features that you might otherwise have to build out yourself like map and location features, cloud backups, sign-in services and more. You can check them all out here.
- API Guides: Google services are set apart from the regular APIs, which you can also read about here. These range from code to create basic animations, to reading sensors and connecting to the internet. There’s tons of info here to add functionality to your app.
- Sample Code: Sometimes it helps to see how someone else did it before you. This section shows you samples of code for various functions. This can help you see how something works, or just use it in your app so you don’t have to reinvent the wheel.
Android Design Guidelines
The counterpart to the developer guidelines is the Design Guidelines. Google is focusing increasingly on teaching its developers how to make apps that not only work well but look good. As such, that means a lot of the work has been done for you to cover the basics like buttons and simple animations.
The place to go to get more info on this is the Android Design Guidelines, which are a second major subsection of Google’s official documentation. Keep in mind that these are here for people who may not have a great grasp on visual design as it relates to creating application interfaces. In other words, if you already know what your app is going to look like, you might not need this. If you already know what you’re app looks like but you’re not good at making apps look good, check this out.
Here is a list of some the helpful areas to start:
- Devices: Android targets more than just phones. This section will help you learn how phones, tablets, TVs and watches all relate and how you can design an interface that adapts to all of them.
- Patterns: Android is built on structured interfaces. This section teaches the building blocks of how apps work so you can design the framework that you’ll be building your design on top of.
- Material Design Documentation: This is technically a separate section for now, but Google’s newest version of Android will introduce a new type of design language called Material Design. Here you can peruse what that means and how to think about designing apps that fit these guidelines. It’s also helpful if you’re not experienced with thinking about how users interact with apps, even if you don’t follow the specific recommendations.
GitHub/BitBucket
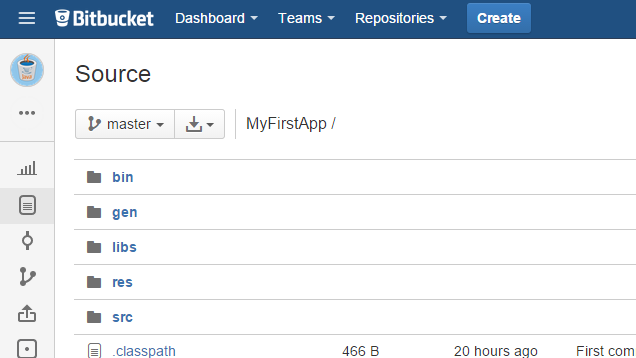
While you’re developing an app, there are a lot of files to manage and you’ll need a way to track changes. Git is one of the most commonly used protocols to manage new versions or changes to existing software. Necessarily, it’s a little more complicated than a basic backup tool. It’s flexible enough to allow you to manage multiple different branches of your app as well as pull from older versions if something goes wrong.
Two of the most common services for managing projects with Git are Github and Bitbucket. Both use the same underlying protocol and can be integrated directly into either Eclipse or Android Studio. BitBucket allows you to have some private repositories (read: storage for projects) without paying money, while GitHub’s free offerings require repositories to be publicly listed unless you pay a little extra. Here are some resources that can help you get started with Git:
- BitBucket Tutorials: Atlassian, the maker of BitBucket, has a series of guides on how to get started with BitBucket and import your projects here. In my personal experience setting up both BitBucket and GitHub, this service and these guides were much easier for the uninitiated to get started with.
- GitHub Guides: GitHub similarly has some tutorials on how to set up its service that you can find here. Some of the guides refer to older versions of the software in some cases, but generally you should be able to get up and running with these.
- Vogella Git Tutorial: Vogella has yet another great tutorial here explaining what Git itself is and how it can help you manage your entire project. While version management is Git’s primary function, there’s a lot more here that Vogella can walk you through.
Developing for Android is far more than just putting Java in a text editor. If you have a little bit of experience with writing code but haven’t dived head first into actual app development yet, there’s a lot you may not be aware you need to know just yet. The good news is, you’re not the first person to go down this road. These are just some of the tools you need and hopefully these guides will put you on the right path.
Cheers
Lifehacker
Got your own question you want to put to Lifehacker? Send it using our [contact text=”contact form”].
Comments
7 responses to “Ask LH: I Want To Write Android Apps, Where Do I Start?”
No mention of MIT’s App Inventor?
http://appinventor.mit.edu/explore/
If you want to just dive in and code, I suggest PhoneGap. I have a strong background in CSS, HTML and Javascript, but very little in Java. You need node.js, Ant and ADB to run it, but you can develop semi-native apps using HTML5, JS and CSS. These apps are basically running in a webbrowser but can access native things like accelerometer, camera and do push notifications.
I’m writing my first app (a tech demo for work that displays news and events from our website) and it’s pretty easy. I’ll wind up learning to code native apps, but while I get my head around how Android actually operates, PhoneGap / Cordova is a fantastic set of tools to get started
Highly recommend against this. Performance is awful, particularly when scrolling through a list.
Had to maintain and bring a pair of old apps up to speed which ran on Cordova, and it was painful. To be fair, my knowledge of HTML/JS/CSS was limited (and the JS was actually Coffeescript), but in the time it took me to figure out how everything worked with the Cordova framework, I could’ve rebuilt the app in native.
So far performance has been excellent for me on my Nexus 5 (natch). Granted my longest list has about 100 items in it (list of calendar events) and I’m using Chocolate Chip UI to handle the vast majority of my UI stuff, but it feels sturdy enough to where I could retrieve the entire calendar (> 10,000 events) and have this thing chugging along nicely.
I’d need to test it on my Android 2.4 device to be sure though.
Make sure you download and install the HAXM accelerator (see https://software.intel.com/en-us/android/articles/speeding-up-the-android-emulator-on-intel-architecture), as the default emulator speed is pretty shocking.
I’d also suggest looking at github for open source projects that you can contribute to. Talk to the main contributors and find out what simple features need to be developed; you’ll often get good advice and help as well as the necessary code reviews to help you write better code.
I’d like to ask: is it possible to cut the umbilical cord?
Can I create an Android app using JUST an Android?
My old PC had its own compiler. A Mac doesn’t need another Mac. Linux machines upport themselves, too. With android being based on Linux, I would have thought there’d be oodles of compilers etc. available…